However, with mutable objects like lists, for example, it is not always the case, depending on how you modify your class attribute. What are the differences? Theyre handy when you need them to look like eager attributes, but you want them to be lazy. To define a class attribute, you place it outside of the. Pythons property() provides a quick and reliable tool for dealing with input data validation. If, for example, function returns current time stamp, in the Using properties in those situations can make your code: Unless you need something more than bare attribute access, dont write properties. Because you need to make sure that every value provided as a radius, including the initialization value, goes through the setter method and gets converted to a floating-point number. what do you mean by saying circular?? For example, you can make your getter method raise an exception every time a user accesses the underlying attribute value. As on of the commenters (Pedro) pointed out and I agree with him, it is much better to set them in the __init__ method. The setter method converts the input value for the radius and assigns it to the non-public ._radius, which is the variable you use to store the final data. Check out the section on the raise statement in the documentation for more information about this topic. Related Tutorial Categories: The new Circle implementation works the same as the example in the section above: You dont need to use a pair of parentheses for calling .radius() as a method. The affectation added a new instance attribute to the object fooand only to that object which is why in the previous example the object barkept printing the class attribute. One should be aware that, because of this, value assigned to class or Join us and get access to hundreds of tutorials, hands-on video courses, and a community of expert Pythonistas: Whats your #1 takeaway or favorite thing you learned? Python doesnt have private variables so-to-speak, but another interesting relationship between class and instance naming comes with name mangling. Why do you need to do that? In fact, I wasnt sure of the answers myself. Because of that, .name lost the rest of the functionality from the base class.
Here is what I have: Doing so ensures that you never save the plaintext password in any retrievable attribute. Finally, the decorator syntax reassigns the new property to the .radius class-level name. Great read! Free Class Irvine Sepetember 7: https://www.getdrip.com/forms/45693356/submissions/new But when carelessly thrown into a given class, theyre sure to trip you up. Heres how you can work with Point in your code: With .get_x() and .get_y(), you can access the current values of ._x and ._y. To prevent unexpected behaviors, you need to think of the mutability of the input data. Heres how it works: When you access .diameter, you get its computed value.
When a mutable class attribute is modified by an object, it does not mutateto turn into an instance attribute for that object. Hold on let's check our namespaces and try to understand what the hell is happening. As the name suggests, a Python namespace is a mapping from names to objects, with the property that there is zero relation between names in different namespaces. In the end, these languages need getter and setter methods because they dont provide a suitable way to change the internal implementation of an attribute if a given requirement changes. This matters big time when using functions for initialization that are dependent on parameters that could change.
You dont have a setter method any longer. Here, class_var is a class attribute, and i_var is an instance attribute: Note that all instances of the class have access to class_var, and that it can also be accessed as a property of the class itself: For Java or C++ programmers, the class attribute is similarbut not identicalto the static member. Well, when you override an existing property from a parent class, you override the whole functionality of that property. Note: If youre worrying about performance at this level, you might not want to be use Python in the first place, as the differences will be on the order of tenths of a millisecondbut its still fun to poke around a bit, and helps for illustrations sake. In that case, you can compute the value just once: Even though this implementation of Circle properly caches the computed diameter, it has the drawback that if you ever change the value of .radius, then .diameter wont return a correct value: In these examples, you create a circle with a radius equal to 42.0. I did not undestand the "Handling assignment" part. (Note: this isnt the exact code (which would be setattr(MyClass, 'class_var', 2)) as __dict__ returns a dictproxy, an immutable wrapper that prevents direct assignment, but it helps for demonstrations sake). Over 2 million developers have joined DZone. (0, 0, 0) Theyre functions that take another function as an argument and return a new function with added functionality. Since your users are free to enter any type of data, you need to make sure that your point only accepts numbers. The getter method generates log information every time you access .radius in your code. Properties allow you to modify the implementation of instance attributes without changing the public API of the class. A class attribute is an attribute of the class (circular, I know) A namespace is a mapping from names to objects, with the property that there is zero relation between names in different namespaces.
if len(self.data) >= MyClass.limit: Then you implement Circle with a managed attribute .radius.
And I'm wondering: what purposes does that serve? Why we can't access names through the instance variable? This essentially overrides the class variable and turns it into an instance variable available, intuitively, only for that instance. When we assign to Foo(2).y, we do half as many lookups, as we immediately assign to the instance namespace (Foo(2).__dict__[y]). Thats also the reason why property() doesnt follow the Python convention for naming classes. You need to manage this situation in a way other than removing .radius. If I change a python class variable in one instance (myinstance.class_var = 4) this does NOT change it for other instances. Note that even though you change the value of the radius, the diameter stays the same. We could get around this using assignment; that is, instead of exploiting the lists mutability, we could assign our Service objects to have their own lists, as follows: In this case, were adding s1.__dict__['data'] = [1], so the original Service.__dict__['data'] remains unchanged.
There are (few) cases to make for that, but this limit-list is not one of them. There is a subtle detail to note in this new implementation of Circle and its .radius attribute.
Sometimes you have a given computed attribute that you use frequently. You pointing out that updating an instance attribute that doesn't exist would update the class attribute helped me solve what I consider a very weird problem. Free Class Fullerton August 24: https://www.getdrip.com/forms/45787421/submissions/new However, if youre creating an attribute that you use frequently, then computing it every time can be costly and wasteful. The code works just like your earlier implementation. This is your most intersting post and very knowledgable post please keep on posting like this share your knwoledge as much as possible it will help others, It's only valid syntax in standard classes if you use type hinting, because its use is exactly that, type hinting. As a final example of computed attributes, say you have a Point class that uses .x and .y as Cartesian coordinates.
You can compare the namespaces by yourself as proof of the previous behavior.
I can foresee me using class variables efficiently going forward. These methods offer a way to change the internal implementation of your attributes without changing your public API. However, in some sections, youll see it called a class to facilitate the explanation. You say "For Java or C++ programmers, the class attribute is similarbut not identicalto the static member. However, unlike property(), cached_property() doesnt block attribute mutations unless you provide a proper setter method. I too was wrong in that it isnt setting a default value for the instance attribute. At the end of the process, you get a full-fledged property with the getter, setter, and deleter methods.
Edit: as Pedro Werneck kindly pointed out, this behavior is largely intended to help out with subclassing. People coming from another language where the public/private distinction are more prevalent might believe it's a good practice to do that for all their "private" attributes. Great read and great examples!
The decorator approach for creating properties requires defining a first method using the public name for the underlying managed attribute, which is .radius in this case. By continuing to use this site you agree to our, iterate over the garbage collectors list of objects, Python Best Practices and Tips by Toptal Developers, Become More Advanced: Avoid the 10 Most Common Mistakes That Python Programmers Make, The 10 Most Common JavaScript Issues Developers Face, Harness the Power of WordPress Hooks: Actions and Filters Explained, gRPC vs. REST: Getting Started With the Best API Protocol, Code Writing Code: An Introduction to the Theory and Practice of Modern Metaprogramming, Me: Im pretty sure it is.
If not, it then looks in the class namespace and returns the attribute (if its present, throwing an error otherwise). (Remember, though: take care when using mutable values as your defaults.). The decorator syntax consists of placing the name of the decorator function with a leading @ symbol right before the definition of the function you want to decorate: In this code fragment, @decorator can be a function or class intended to decorate func(). You can just give a name (and if you want, a type hint). Removing .units and .cents from your public API to use something like .total_cents would break more than one clients code. However, you should know up front that the decorator approach is more popular in the Python community. Free Class Irvine August 31: https://www.getdrip.com/forms/38503762/submissions/new Additionally, property() was implemented in C to ensure optimal performance. The deleter method calls .clear() on the list of children to remove them all: Here, you first create a root node to start populating the tree. When you access an attribute (instance or class attribute) as a property of an object using the dot convention, it searches first in the namespace of that object for that attribute name. No one really knows when (or how) to use em. This module provides all the functionality you would require for logging your code. Below a simplified version of the lookup function. Stuck to instance attributes entirely, as demonstrated in the introduction.
As further evidence, lets use the Python disassembler: When we look at the byte code, its again obvious that Foo.__init__ has to do two assignments, while Bar.__init__ does just one. Just came across this and spent a good hour with it. At the namespace level all instances of Service are accessing and modifying the same list in Service.__dict__ without making their own data attributes in their instance namespaces. However, your users can still access and mutate .units and .cents in their code and get the same result as before. Then you define three non-public methods: Once you have these three methods in place, you create a class attribute called .radius to store the property object.
One thing I wanted to include but didnt have a natural entrance point.
Unsubscribe any time.
If you check your Circle implementations so far, then youll note that their getter and setter methods dont add any real extra processing on top of your attributes. Thanks! For more information feel free to visit our website at http://www.thedevmasters.com Or contact us directly at 8663401375 or contact@thedevmasters.com. For example, you can turn both .x and .y into properties. To be accurate, class attributes in Python and static attributes in Java or C++ have a lot in common, however, they have behavioral differences that I will highlight in this article. >>> a1, a2 = A(), A() A property is a special type of class member that provides functionality thats somewhere in between regular attributes and methods.
In the following two sections, youll learn how to use both approaches.
Instead, use the @property decorator. We assign to Bar.y just once, but instance_of_Foo.y on every call to __init__. In this situation, property() can be an excellent option to keep your current API unchanged. Heres an example that implements a tree node that uses property() to provide most of its functionality, including the ability to clear the list of children of the node at hand: In this example, TreeNode represents a node in your custom tree data type. >>> A.cv, a1.cv, a2.cv This is sort of specific, but I could see a scenario in which you might want to access a piece of data related to every existing instance of a given class. Very well written! The team members who worked on this tutorial are: Master Real-World Python Skills With Unlimited Access to RealPython. If we find, in one class, both an instance attribute and a class attribute with the same name, the access to that name from your object will get you the value in the object namespace. To understand whats happening here, lets talk briefly about Python namespaces. Note: Im on a MacBook Pro with OS X 10.8.5 and Python 2.7.2. The author demonstrates in the "Mutability" section that if the class variable is a mutable object, then all instances see the change even if it was changed from within one instance (myinstance.class_var.append(4)) The class initializer, .__init__(), takes radius as an argument and stores it in a non-public attribute called ._radius. To give Circle a try, run the following code in your Python shell: The .radius property hides the non-public instance attribute ._radius, which is now your managed attribute in this example. In the example, the getter and setter methods dont perform any further processing with ._x and ._y. Since property() is a built-in function, you can use it without importing anything. You also learned how to create read-only, read-write, and write-only attributes. You cover some very subtle topics and make them perfectly clear! You and your fellow programmers will be able to read that docstring using Pythons help(). However, subsequent tests uncover an unexpected behavior: What happened?
>>> A.cv = 3
instance variable (in __init__) using the same function call might be Just one additional remark regarding "Recall that a classs namespace is If you want to signal that a given attribute or method is non-public, then you have to use the well-known Python convention of prefixing the name with an underscore (_). The getter method raises an AttributeError whenever a user tries to retrieve the current password. Technically, theres nothing that stops you from using getter and setter methods in Python. Help on Circle in module __main__ object:, , , [, 'deleter', , 'getter', 'setter'], b'b\xc7^ai\x9f3\xd2g \x89^-\x92\xbe\xe6', b'\xe9l$\x9f\xaf\x9d b\xe8\xc8\xfcaU\r_', 'validation error while mutating "radius"', 14:48:59: "radius" was accessed. You can also specify a way to handle attribute deletion and provide an appropriate docstring for your properties.
Logging can also help you identify sources of problematic data input, analyze the performance of your code, spot usage patterns, and more. Note: The first three arguments to property() take function objects.
Now suppose that you have an important user that comes to you with a new requirement. Here it is:
Heres an example of handling passwords with a write-only property: The initializer of User takes a username and a password as arguments and stores them in .name and .password, respectively. However, there are some things which I would like to clarify. This turns .password into a write-only attribute: In this example, you create john as a User instance with an initial password. If you try to set variable name "x" as a class attribute without "=" or ":", it will raise NameError: name "x" is not defined. Heres an example of how to use property() to create a computed attribute .area in a Rectangle class: In this example, the Rectangle initializer takes width and height as arguments and stores them in regular instance attributes. Finally, you define two getter methods and decorate them with @property. Other than that, both behave as though they exist as separate variables in each instance of the class. Heres how this code works in practice: When it comes to providing computed or lazy attributes, property() is a pretty handy tool. All Rights Reserved. One of those things could be subclassing them to customize their functionalities. I was asked to implement a certain API, and chose to do so in Python. This leads to something interesting though: when you're defining a dataclass, you don't have to set a value for the attribute.
For example: At the namespace level were adding the class_var attribute to foo.__dict__, so when we lookup foo.class_var, we return 2. When assignment (myinstance.class_var = 4) is used to we see the modified class variable isolated to the instance from which it was changed.
The read-only property .area computes and returns the area of the current rectangle every time you access it. Your user will access and mutate them directly in their code. We want to keep track of all the names that have been used. I took a deep breath and started typing. Behind the scenes, it is a game of namespaces. Join the DZone community and get the full member experience. Python class attributes. The mechanism to define the deleter method is similar. The getter method computes the diameter using the radius.
(3, 2, 3) That compliment means a lot--much appreciated.
The most popular approach is to turn your attributes into properties. Leave a comment below and let us know. Circle also implements a .diameter attribute as a property. # Non-public attributes are still accessible, 'Circle' object has no attribute '_radius'. In the following sections, youll code a few examples that will help you get a better practical understanding of common use cases of property(). However, property is a class designed to work as a function rather than as a regular class. Its important to note that assigning the .x and .y properties directly in .__init__() ensures that the validation also occurs during object initialization. Finally, you should avoid writing explicit getter and setter methods and then wrapping them in a property. If you have already read the Zen of Python, the last line states: "Namespaces are one honking great idea -- let's do more of those!" The initializations of Bar are faster by over a second, so the difference here does appear to be statistically significant. Thank you for this article. Not at all. 140178214034336
It stays shared between all the objects of the class with the new elements appended to it. How are you going to put your newfound skills to use? Lets go back to the Service I defined earlier and see how my use of a class variable could have led to problems down the road.
A very wonderful guide for those students who wanted to improve their skills and knowledge about this kind of class.
Thats the reason behind the naming of the attributes ._x and ._y.
. (1, 1, 1) I would like to invite you to join our upcoming Python and Data Science Activities. In Python, a namespace is a mapping between objects and names. ?
Instead of using @property again, you use @radius.setter. You want to provide polar coordinates for your point so that you can use them in a few computations. For example: At the namespace level were setting MyClass.__dict__['class_var'] = 2. Current value: 100, 14:49:24: "radius" was accessed. >>> a1.cv, a2.cv, A.cv In other words, they allow you to create methods that behave like attributes.
Class attributes are tricky, but lets look at a few cases when they would come in handy: Storing constants. This exception allows you to customize the way you implement your immutable Point class. Methods are functions attached to a given class. Pythons property() can also work as a decorator, so you can use the @property syntax to create your properties quickly: This code looks pretty different from the getter and setter methods approach. Heres a summary of what each argument does: The return value of property() is the managed attribute itself. I'm quite frankly amazed you were able to write this much on class variables! This implementation of Point uncovers a fundamental weakness of property(). If you ever need to modify how you compute a given public attribute, then you can turn it into a property.
Say youre working on a Circle class. Now you have two read-only properties, .x and .y, as your coordinates: Here, point.x and point.y are bare-bone examples of read-only properties. This function allows you to turn class attributes into properties or managed attributes. Good job! >>> A.cv = 1 Pythontutorial.net helps you master Python programming from scratch fast. So I did some digging. This way, you can handle the internal implementation for that attribute without exposing getter and setter methods in your API. If nothing is found there as well, it raises an AttributeError.
Charlie (BCS, Princeton) has been an engineering lead at Khan Academy, then Cedar, and nowadays does ML at Spring Discovery. When you define a class in an object-oriented programming language, youll probably end up with some instance and class attributes. Well see how they differ later.
You can think of a function object as the function name without the calling pair of parentheses. If you check Circle again, youll note that you already did so by adding a docstring to the getter method on line 9. Note: If you want to better understand the internal implementation of property as a class, then check out the pure Python Property class described in the documentation.
Here is what I have: Doing so ensures that you never save the plaintext password in any retrievable attribute. Finally, the decorator syntax reassigns the new property to the .radius class-level name. Great read! Free Class Irvine Sepetember 7: https://www.getdrip.com/forms/45693356/submissions/new But when carelessly thrown into a given class, theyre sure to trip you up. Heres how you can work with Point in your code: With .get_x() and .get_y(), you can access the current values of ._x and ._y. To prevent unexpected behaviors, you need to think of the mutability of the input data. Heres how it works: When you access .diameter, you get its computed value.
When a mutable class attribute is modified by an object, it does not mutateto turn into an instance attribute for that object. Hold on let's check our namespaces and try to understand what the hell is happening. As the name suggests, a Python namespace is a mapping from names to objects, with the property that there is zero relation between names in different namespaces. In the end, these languages need getter and setter methods because they dont provide a suitable way to change the internal implementation of an attribute if a given requirement changes. This matters big time when using functions for initialization that are dependent on parameters that could change.
You dont have a setter method any longer. Here, class_var is a class attribute, and i_var is an instance attribute: Note that all instances of the class have access to class_var, and that it can also be accessed as a property of the class itself: For Java or C++ programmers, the class attribute is similarbut not identicalto the static member. Well, when you override an existing property from a parent class, you override the whole functionality of that property. Note: If youre worrying about performance at this level, you might not want to be use Python in the first place, as the differences will be on the order of tenths of a millisecondbut its still fun to poke around a bit, and helps for illustrations sake. In that case, you can compute the value just once: Even though this implementation of Circle properly caches the computed diameter, it has the drawback that if you ever change the value of .radius, then .diameter wont return a correct value: In these examples, you create a circle with a radius equal to 42.0. I did not undestand the "Handling assignment" part. (Note: this isnt the exact code (which would be setattr(MyClass, 'class_var', 2)) as __dict__ returns a dictproxy, an immutable wrapper that prevents direct assignment, but it helps for demonstrations sake). Over 2 million developers have joined DZone. (0, 0, 0) Theyre functions that take another function as an argument and return a new function with added functionality. Since your users are free to enter any type of data, you need to make sure that your point only accepts numbers. The getter method generates log information every time you access .radius in your code. Properties allow you to modify the implementation of instance attributes without changing the public API of the class. A class attribute is an attribute of the class (circular, I know) A namespace is a mapping from names to objects, with the property that there is zero relation between names in different namespaces.
if len(self.data) >= MyClass.limit: Then you implement Circle with a managed attribute .radius.
And I'm wondering: what purposes does that serve? Why we can't access names through the instance variable? This essentially overrides the class variable and turns it into an instance variable available, intuitively, only for that instance. When we assign to Foo(2).y, we do half as many lookups, as we immediately assign to the instance namespace (Foo(2).__dict__[y]). Thats also the reason why property() doesnt follow the Python convention for naming classes. You need to manage this situation in a way other than removing .radius. If I change a python class variable in one instance (myinstance.class_var = 4) this does NOT change it for other instances. Note that even though you change the value of the radius, the diameter stays the same. We could get around this using assignment; that is, instead of exploiting the lists mutability, we could assign our Service objects to have their own lists, as follows: In this case, were adding s1.__dict__['data'] = [1], so the original Service.__dict__['data'] remains unchanged.
There are (few) cases to make for that, but this limit-list is not one of them. There is a subtle detail to note in this new implementation of Circle and its .radius attribute.
Sometimes you have a given computed attribute that you use frequently. You pointing out that updating an instance attribute that doesn't exist would update the class attribute helped me solve what I consider a very weird problem. Free Class Fullerton August 24: https://www.getdrip.com/forms/45787421/submissions/new However, if youre creating an attribute that you use frequently, then computing it every time can be costly and wasteful. The code works just like your earlier implementation. This is your most intersting post and very knowledgable post please keep on posting like this share your knwoledge as much as possible it will help others, It's only valid syntax in standard classes if you use type hinting, because its use is exactly that, type hinting. As a final example of computed attributes, say you have a Point class that uses .x and .y as Cartesian coordinates.
I can foresee me using class variables efficiently going forward. These methods offer a way to change the internal implementation of your attributes without changing your public API. However, in some sections, youll see it called a class to facilitate the explanation. You say "For Java or C++ programmers, the class attribute is similarbut not identicalto the static member. However, unlike property(), cached_property() doesnt block attribute mutations unless you provide a proper setter method. I too was wrong in that it isnt setting a default value for the instance attribute. At the end of the process, you get a full-fledged property with the getter, setter, and deleter methods.
Edit: as Pedro Werneck kindly pointed out, this behavior is largely intended to help out with subclassing. People coming from another language where the public/private distinction are more prevalent might believe it's a good practice to do that for all their "private" attributes. Great read and great examples!
The decorator approach for creating properties requires defining a first method using the public name for the underlying managed attribute, which is .radius in this case. By continuing to use this site you agree to our, iterate over the garbage collectors list of objects, Python Best Practices and Tips by Toptal Developers, Become More Advanced: Avoid the 10 Most Common Mistakes That Python Programmers Make, The 10 Most Common JavaScript Issues Developers Face, Harness the Power of WordPress Hooks: Actions and Filters Explained, gRPC vs. REST: Getting Started With the Best API Protocol, Code Writing Code: An Introduction to the Theory and Practice of Modern Metaprogramming, Me: Im pretty sure it is.
If not, it then looks in the class namespace and returns the attribute (if its present, throwing an error otherwise). (Remember, though: take care when using mutable values as your defaults.). The decorator syntax consists of placing the name of the decorator function with a leading @ symbol right before the definition of the function you want to decorate: In this code fragment, @decorator can be a function or class intended to decorate func(). You can just give a name (and if you want, a type hint). Removing .units and .cents from your public API to use something like .total_cents would break more than one clients code. However, you should know up front that the decorator approach is more popular in the Python community. Free Class Irvine August 31: https://www.getdrip.com/forms/38503762/submissions/new Additionally, property() was implemented in C to ensure optimal performance. The deleter method calls .clear() on the list of children to remove them all: Here, you first create a root node to start populating the tree. When you access an attribute (instance or class attribute) as a property of an object using the dot convention, it searches first in the namespace of that object for that attribute name. No one really knows when (or how) to use em. This module provides all the functionality you would require for logging your code. Below a simplified version of the lookup function. Stuck to instance attributes entirely, as demonstrated in the introduction.
As further evidence, lets use the Python disassembler: When we look at the byte code, its again obvious that Foo.__init__ has to do two assignments, while Bar.__init__ does just one. Just came across this and spent a good hour with it. At the namespace level all instances of Service are accessing and modifying the same list in Service.__dict__ without making their own data attributes in their instance namespaces. However, your users can still access and mutate .units and .cents in their code and get the same result as before. Then you define three non-public methods: Once you have these three methods in place, you create a class attribute called .radius to store the property object.
One thing I wanted to include but didnt have a natural entrance point.
Unsubscribe any time.
If you check your Circle implementations so far, then youll note that their getter and setter methods dont add any real extra processing on top of your attributes. Thanks! For more information feel free to visit our website at http://www.thedevmasters.com Or contact us directly at 8663401375 or contact@thedevmasters.com. For example, you can turn both .x and .y into properties. To be accurate, class attributes in Python and static attributes in Java or C++ have a lot in common, however, they have behavioral differences that I will highlight in this article. >>> a1, a2 = A(), A() A property is a special type of class member that provides functionality thats somewhere in between regular attributes and methods.
In the following two sections, youll learn how to use both approaches.
Instead, use the @property decorator. We assign to Bar.y just once, but instance_of_Foo.y on every call to __init__. In this situation, property() can be an excellent option to keep your current API unchanged. Heres an example that implements a tree node that uses property() to provide most of its functionality, including the ability to clear the list of children of the node at hand: In this example, TreeNode represents a node in your custom tree data type. >>> A.cv, a1.cv, a2.cv This is sort of specific, but I could see a scenario in which you might want to access a piece of data related to every existing instance of a given class. Very well written! The team members who worked on this tutorial are: Master Real-World Python Skills With Unlimited Access to RealPython. If we find, in one class, both an instance attribute and a class attribute with the same name, the access to that name from your object will get you the value in the object namespace. To understand whats happening here, lets talk briefly about Python namespaces. Note: Im on a MacBook Pro with OS X 10.8.5 and Python 2.7.2. The author demonstrates in the "Mutability" section that if the class variable is a mutable object, then all instances see the change even if it was changed from within one instance (myinstance.class_var.append(4)) The class initializer, .__init__(), takes radius as an argument and stores it in a non-public attribute called ._radius. To give Circle a try, run the following code in your Python shell: The .radius property hides the non-public instance attribute ._radius, which is now your managed attribute in this example. In the example, the getter and setter methods dont perform any further processing with ._x and ._y. Since property() is a built-in function, you can use it without importing anything. You also learned how to create read-only, read-write, and write-only attributes. You cover some very subtle topics and make them perfectly clear! You and your fellow programmers will be able to read that docstring using Pythons help(). However, subsequent tests uncover an unexpected behavior: What happened?

instance variable (in __init__) using the same function call might be Just one additional remark regarding "Recall that a classs namespace is If you want to signal that a given attribute or method is non-public, then you have to use the well-known Python convention of prefixing the name with an underscore (_). The getter method raises an AttributeError whenever a user tries to retrieve the current password. Technically, theres nothing that stops you from using getter and setter methods in Python. Help on Circle in module __main__ object:
Logging can also help you identify sources of problematic data input, analyze the performance of your code, spot usage patterns, and more. Note: The first three arguments to property() take function objects.
Now suppose that you have an important user that comes to you with a new requirement. Here it is:
Heres an example of handling passwords with a write-only property: The initializer of User takes a username and a password as arguments and stores them in .name and .password, respectively. However, there are some things which I would like to clarify. This turns .password into a write-only attribute: In this example, you create john as a User instance with an initial password. If you try to set variable name "x" as a class attribute without "=
For example: At the namespace level were adding the class_var attribute to foo.__dict__, so when we lookup foo.class_var, we return 2. When assignment (myinstance.class_var = 4) is used to we see the modified class variable isolated to the instance from which it was changed.
The read-only property .area computes and returns the area of the current rectangle every time you access it. Your user will access and mutate them directly in their code. We want to keep track of all the names that have been used. I took a deep breath and started typing. Behind the scenes, it is a game of namespaces. Join the DZone community and get the full member experience. Python class attributes. The mechanism to define the deleter method is similar. The getter method computes the diameter using the radius.
(3, 2, 3) That compliment means a lot--much appreciated.
The most popular approach is to turn your attributes into properties. Leave a comment below and let us know. Circle also implements a .diameter attribute as a property. # Non-public attributes are still accessible, 'Circle' object has no attribute '_radius'. In the following sections, youll code a few examples that will help you get a better practical understanding of common use cases of property(). However, property is a class designed to work as a function rather than as a regular class. Its important to note that assigning the .x and .y properties directly in .__init__() ensures that the validation also occurs during object initialization. Finally, you should avoid writing explicit getter and setter methods and then wrapping them in a property. If you have already read the Zen of Python, the last line states: "Namespaces are one honking great idea -- let's do more of those!" The initializations of Bar are faster by over a second, so the difference here does appear to be statistically significant. Thank you for this article. Not at all. 140178214034336
It stays shared between all the objects of the class with the new elements appended to it. How are you going to put your newfound skills to use? Lets go back to the Service I defined earlier and see how my use of a class variable could have led to problems down the road.
A very wonderful guide for those students who wanted to improve their skills and knowledge about this kind of class.
Thats the reason behind the naming of the attributes ._x and ._y.
. (1, 1, 1) I would like to invite you to join our upcoming Python and Data Science Activities. In Python, a namespace is a mapping between objects and names. ?
Instead of using @property again, you use @radius.setter. You want to provide polar coordinates for your point so that you can use them in a few computations. For example: At the namespace level were setting MyClass.__dict__['class_var'] = 2. Current value: 100, 14:49:24: "radius" was accessed. >>> a1.cv, a2.cv, A.cv In other words, they allow you to create methods that behave like attributes.
Class attributes are tricky, but lets look at a few cases when they would come in handy: Storing constants. This exception allows you to customize the way you implement your immutable Point class. Methods are functions attached to a given class. Pythons property() can also work as a decorator, so you can use the @property syntax to create your properties quickly: This code looks pretty different from the getter and setter methods approach. Heres a summary of what each argument does: The return value of property() is the managed attribute itself. I'm quite frankly amazed you were able to write this much on class variables! This implementation of Point uncovers a fundamental weakness of property(). If you ever need to modify how you compute a given public attribute, then you can turn it into a property.
Say youre working on a Circle class. Now you have two read-only properties, .x and .y, as your coordinates: Here, point.x and point.y are bare-bone examples of read-only properties. This function allows you to turn class attributes into properties or managed attributes. Good job! >>> A.cv = 1 Pythontutorial.net helps you master Python programming from scratch fast. So I did some digging. This way, you can handle the internal implementation for that attribute without exposing getter and setter methods in your API. If nothing is found there as well, it raises an AttributeError.
Charlie (BCS, Princeton) has been an engineering lead at Khan Academy, then Cedar, and nowadays does ML at Spring Discovery. When you define a class in an object-oriented programming language, youll probably end up with some instance and class attributes. Well see how they differ later.
You can think of a function object as the function name without the calling pair of parentheses. If you check Circle again, youll note that you already did so by adding a docstring to the getter method on line 9. Note: If you want to better understand the internal implementation of property as a class, then check out the pure Python Property class described in the documentation.
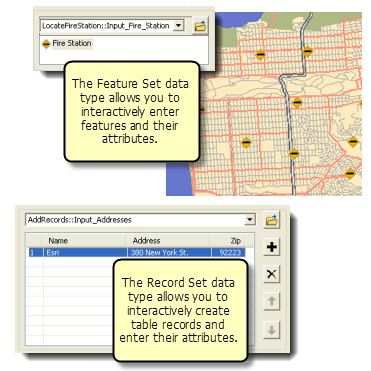
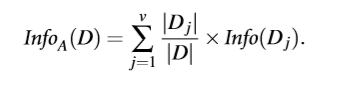