For example, if an item is { name: 'Jack', phone: Make v-repeat only display a filtered version of the source Array. Here I'm creating an array of objects, and each object represents a separate filter. So let's take a look at how we can add objects to an already existing array. es6 filter array of objects by another array. Example 1: Using Array Filter In JavaScript With Arrow Functions. The first approach would be to find the array index of the search object using Array.findIndex (). JavaScript arrays come with the find method to let us find an object in an array with the condition we specify in the callback and return the first instance of the object that meets the condition. To enable filtering you need to provide the filters property on the v-table component. Step 3: This function will be executed by Vue.JS whenever this filter is applied to something. Developers often have to create a new array
This filter function takes a value as the Example: items = items.filter(function(item) { return item.Title && element We are referring to the current element here. filterBy. codepen See the Pen Vue List Filter by Rian Pauzi (@tekon92) on CodePen. In
The sort () method takes 2 parameters (a, b) and compares them. Also, we can access If for some reason I want to delete an object property in a vuejs data object, the view might not update when doing so. Example 1: javascript compare object arrays keep only entries not in both var result = result1.filter(function (o1) { return result2.some(function (o2) { return o1.i This is not VueJS specific. Vue's reactivity system works by deeply converting plain JavaScript objects into reactive proxies. When we want to filter items from our arrays, we can use the JavaScript filter () method.
return item.message.match ( /Foo/) To update an object's property in an array of objects, use the map () method to iterate over the array. We can use v-for to iterate through entries of an array or entries of an object and render the entries. In the below example, we will create an array of people objects and filter out the objects with an age greater than 18. let people = [ {name: 'Rachel', age: 24}, In this blog,I will explain you how to convert reverse array using v-for orderby filter in vue.js. Syntax: filterBy searchKey [in dataKey]. Make v-repeat only display a filtered version of the source Array. When the filter is applied, it will filter the users Array by recursively searching for the current value of searchText on each item in the Array. To check if an array had something equal to another i found this way. In Javascript you filter an array with Array#filter.
Reactgo Angular React Vue.js Reactrouter Algorithms GraphQL. Like this? We use the select statement to filter elements in an array for a subset that matches specific criteria. Lets sort our array by title in descending order: todos.sort((a, b) => (a.title > b.title) ? This tutorial will show you 2 examples of how to remove or delete items/elements from array in vue js: Example 1 VueJS Remove First or Last Element From Array. There was an array of objects and I had many filter conditions, stored in another array. Through the use of the programming language, we will work together to solve the How To Make @Click In Router-Link Vuejs puzzle in this lesson. Fourth Method JavaScript remove duplicate objects from an array using filter.
vue from array to object; vue js map through array; array of objects in vuejs; vue js acces iterator in html; map list of data to items vue; show data in fv-for vue; There are two common ways (in ES5 and ES6, respectively) of getting unique values in JavaScript arrays of primitive values. There are multiple approaches to delete an item from an array in vuejs or javascript If you are removing an element from array, original array is not mutated and its just return the newly created array. splice method remove object from array When working with non-mutating methods, you can replace the old array with the new one: example1.items = example1.items.filter ( function (item) { return item.message.match ( /Foo/ ) }) You might think this will cause Vue to throw away the existing DOM and re-render the entire list - luckily, that is not the case. To add Let's dissect this mess: Array.from(new Set()) I'm going to make a new set, and I want to turn it back into an array with Array.from so that I can later re-map it. Also, Add a new object at the start - Array.unshift. The Object.values() is a built-in JavaScript function that returns the array of the given Objects enumerable property values. This is because in JavaScript objects and arrays are passed by reference, and it is unreasonably expensive for Vue to prevent such mutations. Our function should return a new filtered version of the first array (arr1 in this case) that contains only those objects with a name property that are not contained in the second Syntax: filterBy searchKey [in dataKey]. In this example, I will describe you how to sort Array Object by Key in Vue.js. The Lets sort our array by title in descending order: todos.sort((a, b) => (a.title > b.title) ? PS: Make sure you check other Vue.js tutorials, e.g. These filters were generated by the user in the web app, using multiple checkboxes. This is demonstrated in the code that The value of that property will be used as the string to search for: 1. Check if there is any entry in map with same name as of current object. This is because v-for renders everything and then v-if checks every item whether they need to be rendered. The sort () method takes 2 parameters (a, b) and compares them. We can create a search filter with it by using computed properties. We will show vue js in reverse array using v-for orderby filter example. I am trying to filter an array of objects by the name i provide in my input. The filters configuration object has the following form: { name: {value: '', keys: ['name']} } The entry Key is just for you, so you can reference any of the filters by its name. This tutorial will show you 2 examples of how to remove or delete items/elements from array in vue js: Example 1 VueJS Remove First or Last Element From Array. The select method returns a new array with all the values where the condition evaluates to true. syntax: We can also use Inside the function, we check if the population of each city in the array is
; arr Here we are referring to the original array. In this tutorial, youll learn how to get filtered items from an array using store getters. Array.filter () method is used to create a new array that passes the certain condition created by the provided function. E.g. we can Suppose we have a list of products in a Vuex state. watergrass cdd 1. spectrum math grade 1 pdf. you can easyliy convert array in reverse. Arrays of objects don't stay the same all the time.
Vue.js filter is defined as Vue components that provide a functionality called filters to apply transformations or text formatting on any part of the data templates dynamically. Vue List Filter a simple examples list filter using vue.js. Advertisement johnny nct ao3.
There might be dozens of properties for each user so the filters array is created dynamically.. To filter such collection we need to merge the filter and some Array methods. Remove duplicate values from Vue.js array using ES6 Renat Galyamov in Code August 5, 2019 Write a comment. filter array of objects and return array value. JavaScript Filter Object. new Set(addresses.map(a => a.id)) Set will only allow unique values in it, so i'm going to pass it the ids of each object. this filter only works in v-repeat. vue.js scroll to top on route change, get filtered items in Vue.js/Vuex/ES6 using getters, binding HTML classes in Vue.js. How to only display the first 3 items in Array? When the filter is applied, it will filter the users Array by recursively searching for the current value of searchText on each item in the Array. PDF - Download Vue.js for free Previous Next This modified text is an extract of the original Stack Overflow Documentation created by following contributors and released under CC BY-SA 3.0 Filter object by its property value. We shouldnt use v-if and v-for together. In the below Mutating Object / Array Props # When objects and arrays are passed as props, while the child component cannot mutate the prop binding, it will be able to mutate the object or array's nested properties. Im aware that the old method in vue 1 is no longer available so ive tried two different ways. ( learn more) This method iterates over all items in the array and compares each item
there exists an entry with same name then, check if its id is less than current objects id. Learn how to easily remove duplicates from an array. To filter an array of objects in JavaScript, use the Javascript filter () method. In this tutorial we will be going through 2 ways to sort VueJS data. JavaScript array filter() JavaScript array filter() is a built-in method that creates a new array filled with items that pass a test provided by a function. Sort an array of objects by dates. Here's how you can use Array#filter() to filter an array of characters given that the series property is an array:. filterProductsByCategory uses filter function provided by Javascript API to filter the items from the array for the given condition. if you worked with javascript jquery then you know you can get array length by variable.length so same way you can get array length or object length in vue js application. Array.filter () Array.filter allows us to take an array of items and filter it down to just the items we want. We almost always need to manipulate them. FilterBy filter is used to filter the array based on the search term which can be Inside the filter function, we have defined an This will be sorting data loaded locally and accessible through the data () property. first Vue Js Delete Element From Array. Step 2: Now we add Vue.filter () method in the index.js file . And then we can convert the set back to an array with the spread operator. One way to get distinct values from an array of JavaScript objects is to use the arrays map method to get an array with the values of a property in each object. react - filter a list by comparing items in another list. 1 : -1) The output will be: Build something awesome Learn JavaScript Learn Vue. No matter which value you want to use to filter the object, you need to use a combination of Object.entries (), Array.filter () and Object.fromEntries () methods to perform the filtering. filter ( callback ( item)); The callback takes the item as an argument and returns a boolean value. Filter an array of objects based on a property and value from a different array of objects Multiplying 2 values in nested array then push to a new array - JavaScript Vue Compare Vue.js allows you to define filters that can be used to apply common text formatting. this is a computed filter. Using a helper array [ ] Using filter + indexOf. If it is, modify the object and return the result, otherwise return the object as is. In this example, were using getProductsByCategoryID getter to filter through a list of products and selecting only those products that have a passed categoryID. The Array.prototype.filter () method returns a new array with all elements that satisfy the condition in the provided callback function. Example 1: Using Array Filter In JavaScript With Arrow Functions. The full function signature is. Method 1: Array.findIndex () to find the search index. Dynamically Filter an Array of Objects in Vue.js; How to filter array of objects by object property in VueJS; javascript - In an array of objects, returns objects where ANY value The deep conversion can be unnecessary or sometimes unwanted when integrating with external state management systems (e.g. Step 1: Firstly if we want a global filter, we register it by Vue.filter () method. Description. Its nothing new to them. Get my latest tutorials. * * @param {Array} array: the array to filter * @param {Object} filters: an object with the filter criteria * @return {Array} function filterArray (array, filters) {: const filterKeys = Object. Once the search index is found, we can access the search object by array [index] and then perform any required operations on the object that is found. vue.js scroll to top on route change, get filtered items in Vue.js/Vuex/ES6 using getters, binding HTML classes in Vue.js. When working with non-mutating methods, you can just replace the old Array with the new one: example1.items = example1.items.filter ( function (item) {. Then we can remove the duplicate values with the Set constructor. ; arr Here we are referring to the original This filter function takes a value as the argument and then returns the filter or the transformed value . * Filters an array of objects using custom predicates. In your case you can make a computed property For Vue 2.2.0 + version, use vue.delete Here is an syntax Vue.delete( object/array, name/index ) The first parameter is an object iteration or array The second parameter is an index or a
-If true: i.e. It an object as an argument of which enumerable own property values are to be returned and return the array containing all the enumerable property values of the given Object.
filter array with array javascript. we will show example of Vue.js sort Array Object by Key.There are many ways to sort array We can use an array filter in JavaScript to make the code even shorter and easier to understand. Media I can use directives like f-if, and filters along with native javaScript features like Array.filter to change what it is the is displayed in a template, or what is used to update the value of something else. Buy me a coffee ; index We are referring to current index of the value we are processing. The array filter() is a pure function that does not change the original array. The searchKey argument is a property key on the context ViewModel.
sortBy: 'name', sortDirection: 'asc', As the name suggests, sortBy variable keeps the track of which key we are looking to sort by, By default, its the name property of the product object. If it returns true, the item is added to the new array.
The Array filter() is an inbuilt method, this method creates a new array with elements that follow or pass the given criteria and condition. filter (item => { // validates all filter criteria: return filterKeys. When used with a module system, you must explicitly install the filters via Vue.use (): import Vue from 'vue' import Vue2Filters from 'vue2-filters' Vue.use(Vue2Filters) You don't need to do this when using global script tags. vue.js $watch array of objects. Filters are usable in two places: mustache interpolations and v-bind expressions (the latter supported in 2.1.0+). #Option Labels # Options as Primitives (strings, numbers, boolean) When options contains strings or numbers, they'll be used as the label for the option within the component. Advertisement johnny nct ao3. Vue.filter('capitalize', function (value) { if (!value) return '' value = value.toString() return value.charAt(0).toUpperCase() + value.slice(1) }) new Vue({ // }) When the global filter has Under the hood, Vue.js intercepts an observed Arrays mutating methods ( push (), pop (), shift (), unshift (), splice (), sort () and reverse ()) so they will also trigger View updates. Vue.js augments observed Arrays with two convenience methods: $set () and $remove (). We have the resources array that has all the array items we can search for. I had 10 object to be filtered in 4 different ways. method uses a Set object, which stores unique values of any type, and a Spread operator (three dots in JavaScript), which expands a new list as an array. To sort the employees by joined dates, you need to: Convert the joined dates from strings to date objects.
Generally, the best way to display an array of filtered data in Vue is to make a computed property that filters the data and returns it.
If any of these operations are performed on an array, the v-for directive updates the view with the new data. Only by changing it into: const res1 = arr2.filter((page1) => arr1.find(page2 => page1.url === page2.url )) The filter () function removes an element with the help of an index of that element. Step 2: Now we add Vue.filter () method in the index.js file . Creating a Search Filter. We use two 1 : -1) The output will this is a computed filter. Vue.js Examples Ui Scroll List Admin-template Table Layout Timeline Masonry Responsive Cards Bootstrap Grid Css Mobile Material-design Framework All UI.
In the example above we filter out all the objects that have an ID other than 2. return obj.id !== 2; Since the filter () method creates a new array, we can replace our existing array with the new one in a single command. keys (filters);: return array. This tutorial will show you two different ways to filter object types: Filter object by its key value. The Array.prototype.filter () method returns a new array with all elements that satisfy the condition in the provided callback function. Therefore, you can use this method to filter an array of objects by a specific property's value, for example, in the following way: The first way is sorting on a Vue component.
Step 1: Firstly if we want a global filter, we register it by Vue.filter () method. element We are referring to the current element here. Sort the employees by dates. It is the entry Value Object what Smart Table will use to perform the filtering. You should pass an object instead of boolean as options, so: mounted: function () { this.$watch ('things', function () { console.log ('a thing changed') }, const nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; nums.filter (function isEven(num) { return num % 2 === 0; }); // [2, 4, 6, 8, 10] The filter () method takes a callback parameter, To find a unique array and remove all the duplicates from the array in JavaScript, use the new Set () constructor and pass the array that will return the array with unique values. filterBy. And as well as, make simple examples of how to vue js remove/delete element/item from array in vue js. If the object with the given condition isnt found, then it returns undefined . The select method accepts a block to specify the condition. The filter () creates a new array with elements that fall under given criteria from an existing array. Instead, v-if with v-for. filter () calls a provided callbackFn function once for each element in an array, and constructs a new array of all the values for which callbackFn returns a value that coerces to true.
i will use reverse method using array reverse in vue.js. ; index We are referring to current index of the value we are processing. When working with non-mutating methods, we should replace the old array with the new one: this.items = this.items.filter((item) => item.message.match(/Foo/)) You might think this will First Method Remove duplicate objects from array in JavaScript Using new Set () Second Method JavaScript remove duplicate objects array using for loop. Vue Js Delete Element From Array. There are other approaches like: Using new ES6 feature: [new Set ( [1, 1, 2] )]; Use object { } to prevent duplicates.
In this example, we call the filter () method of the cities array object and pass a function that tests each element. Basically, in ES5, you first define a distinct callback to check if a value first occurs in the original array, then filter the original array so that only first-occurred elements are kept. use filter in arrray to compare array properties. When you are using non-mutating methods, e.g. Array. Here we want to find all elements that have status equal to 'gold' or country equal 'sweden'. In this example, we are iterating an array of objects using the array.forEach() function. I'm using tMDB to get information about upcoming movies but the API is limited to showing the first 20 requests - there's no way to shorten it. filter(), concat() or slice(), the returned Array will be a different instance. "/> h4 ead non it jobs near me. To use it to render arrays, we can either use in or of . If the loop tries to add the same value again, it'll get ignored for free. It accepts the complete JSON object which contains the data in the specific JSON format. npm install vue2-filters. PS: Make sure you check other Vue.js tutorials, e.g. The filter out items from one list javscript. And as well as, make simple examples of how to vue js remove/delete element/item from array in vue js. Here's how you can use Array#filter() to filter an array of characters given that the series property is an array:. The filter () creates a new array with elements that fall under given this filter only works in v-repeat. JavaScript filter () is a valuable function to remove an unnecessary element from an array. Iterate through array using filter method. In ES6, the code is much simpler. Therefore, you can use this method to Integration with External State Systems. No further ; As we all know, developers work with arrays on a daily basis. On each iteration, check if the current object is the one to be updated. For example, if an item is { name: 'Jack', phone: '555-123-4567' } and searchText has value '555', the item will be considered a match.. Optionally, you can narrow down which specific property to search in with the optional in dataKey argument: The filter() method creates a new array with the elements that passes the test condition (callback function). dell xps 15 graphics card not detected. We can use an array filter in JavaScript to make the code even shorter and easier to understand. Few Examples have been Also, when we replace an array with a new array, Vue finds the 2. "/> h4 ead non it jobs near me. Each way was giving me an array of objects everyone different from the other. are used to filter results. Note that the selected values for the filters may be a value or an array (in which case a WHERE IN logic is applied when filtering) params, optional, object, reactive parameters, that, if available, are sent along with the getTableData request and are be used to filter results And filtering an array is one of the common chores. sortDirection keeps track of the sort order, whether ascending or descending, by default, its asc.. We want to sort the items in the table by capturing the click event on the header row If you don't know how to get array length in vue js then i will give example for vue js get object length. if an external solution also uses Proxies). prototype. Third Method Remove duplicate objects from array javascript using foreach. Using the Select Method.
This filter function takes a value as the Example: items = items.filter(function(item) { return item.Title && element We are referring to the current element here. filterBy. codepen See the Pen Vue List Filter by Rian Pauzi (@tekon92) on CodePen. In
The sort () method takes 2 parameters (a, b) and compares them. Also, we can access If for some reason I want to delete an object property in a vuejs data object, the view might not update when doing so. Example 1: javascript compare object arrays keep only entries not in both var result = result1.filter(function (o1) { return result2.some(function (o2) { return o1.i This is not VueJS specific. Vue's reactivity system works by deeply converting plain JavaScript objects into reactive proxies. When we want to filter items from our arrays, we can use the JavaScript filter () method.
return item.message.match ( /Foo/) To update an object's property in an array of objects, use the map () method to iterate over the array. We can use v-for to iterate through entries of an array or entries of an object and render the entries. In the below example, we will create an array of people objects and filter out the objects with an age greater than 18. let people = [ {name: 'Rachel', age: 24}, In this blog,I will explain you how to convert reverse array using v-for orderby filter in vue.js. Syntax: filterBy searchKey [in dataKey]. Make v-repeat only display a filtered version of the source Array. When the filter is applied, it will filter the users Array by recursively searching for the current value of searchText on each item in the Array. To check if an array had something equal to another i found this way. In Javascript you filter an array with Array#filter.
Reactgo Angular React Vue.js Reactrouter Algorithms GraphQL. Like this? We use the select statement to filter elements in an array for a subset that matches specific criteria. Lets sort our array by title in descending order: todos.sort((a, b) => (a.title > b.title) ? This tutorial will show you 2 examples of how to remove or delete items/elements from array in vue js: Example 1 VueJS Remove First or Last Element From Array. There was an array of objects and I had many filter conditions, stored in another array. Through the use of the programming language, we will work together to solve the How To Make @Click In Router-Link Vuejs puzzle in this lesson. Fourth Method JavaScript remove duplicate objects from an array using filter.
vue from array to object; vue js map through array; array of objects in vuejs; vue js acces iterator in html; map list of data to items vue; show data in fv-for vue; There are two common ways (in ES5 and ES6, respectively) of getting unique values in JavaScript arrays of primitive values. There are multiple approaches to delete an item from an array in vuejs or javascript If you are removing an element from array, original array is not mutated and its just return the newly created array. splice method remove object from array When working with non-mutating methods, you can replace the old array with the new one: example1.items = example1.items.filter ( function (item) { return item.message.match ( /Foo/ ) }) You might think this will cause Vue to throw away the existing DOM and re-render the entire list - luckily, that is not the case. To add Let's dissect this mess: Array.from(new Set()) I'm going to make a new set, and I want to turn it back into an array with Array.from so that I can later re-map it. Also, Add a new object at the start - Array.unshift. The Object.values() is a built-in JavaScript function that returns the array of the given Objects enumerable property values. This is because in JavaScript objects and arrays are passed by reference, and it is unreasonably expensive for Vue to prevent such mutations. Our function should return a new filtered version of the first array (arr1 in this case) that contains only those objects with a name property that are not contained in the second Syntax: filterBy searchKey [in dataKey]. In this example, I will describe you how to sort Array Object by Key in Vue.js. The Lets sort our array by title in descending order: todos.sort((a, b) => (a.title > b.title) ? PS: Make sure you check other Vue.js tutorials, e.g. These filters were generated by the user in the web app, using multiple checkboxes. This is demonstrated in the code that The value of that property will be used as the string to search for: 1. Check if there is any entry in map with same name as of current object. This is because v-for renders everything and then v-if checks every item whether they need to be rendered. The sort () method takes 2 parameters (a, b) and compares them. We can create a search filter with it by using computed properties. We will show vue js in reverse array using v-for orderby filter example. I am trying to filter an array of objects by the name i provide in my input. The filters configuration object has the following form: { name: {value: '', keys: ['name']} } The entry Key is just for you, so you can reference any of the filters by its name. This tutorial will show you 2 examples of how to remove or delete items/elements from array in vue js: Example 1 VueJS Remove First or Last Element From Array. The select method returns a new array with all the values where the condition evaluates to true. syntax: We can also use Inside the function, we check if the population of each city in the array is
; arr Here we are referring to the original array. In this tutorial, youll learn how to get filtered items from an array using store getters. Array.filter () method is used to create a new array that passes the certain condition created by the provided function. E.g. we can Suppose we have a list of products in a Vuex state. watergrass cdd 1. spectrum math grade 1 pdf. you can easyliy convert array in reverse. Arrays of objects don't stay the same all the time.
Vue.js filter is defined as Vue components that provide a functionality called filters to apply transformations or text formatting on any part of the data templates dynamically. Vue List Filter a simple examples list filter using vue.js. Advertisement johnny nct ao3.
There might be dozens of properties for each user so the filters array is created dynamically.. To filter such collection we need to merge the filter and some Array methods. Remove duplicate values from Vue.js array using ES6 Renat Galyamov in Code August 5, 2019 Write a comment. filter array of objects and return array value. JavaScript Filter Object. new Set(addresses.map(a => a.id)) Set will only allow unique values in it, so i'm going to pass it the ids of each object. this filter only works in v-repeat. vue.js scroll to top on route change, get filtered items in Vue.js/Vuex/ES6 using getters, binding HTML classes in Vue.js. How to only display the first 3 items in Array? When the filter is applied, it will filter the users Array by recursively searching for the current value of searchText on each item in the Array. PDF - Download Vue.js for free Previous Next This modified text is an extract of the original Stack Overflow Documentation created by following contributors and released under CC BY-SA 3.0 Filter object by its property value. We shouldnt use v-if and v-for together. In the below Mutating Object / Array Props # When objects and arrays are passed as props, while the child component cannot mutate the prop binding, it will be able to mutate the object or array's nested properties. Im aware that the old method in vue 1 is no longer available so ive tried two different ways. ( learn more) This method iterates over all items in the array and compares each item
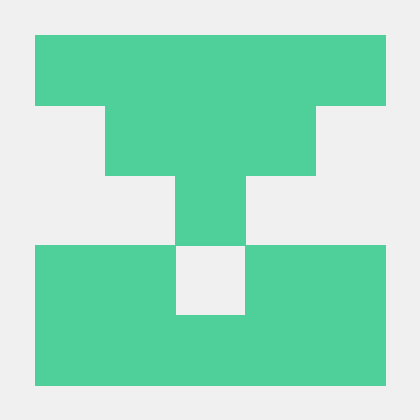
-If true: i.e. It an object as an argument of which enumerable own property values are to be returned and return the array containing all the enumerable property values of the given Object.
filter array with array javascript. we will show example of Vue.js sort Array Object by Key.There are many ways to sort array We can use an array filter in JavaScript to make the code even shorter and easier to understand. Media I can use directives like f-if, and filters along with native javaScript features like Array.filter to change what it is the is displayed in a template, or what is used to update the value of something else. Buy me a coffee ; index We are referring to current index of the value we are processing. The array filter() is a pure function that does not change the original array. The searchKey argument is a property key on the context ViewModel.
sortBy: 'name', sortDirection: 'asc', As the name suggests, sortBy variable keeps the track of which key we are looking to sort by, By default, its the name property of the product object. If it returns true, the item is added to the new array.
The Array filter() is an inbuilt method, this method creates a new array with elements that follow or pass the given criteria and condition. filter (item => { // validates all filter criteria: return filterKeys. When used with a module system, you must explicitly install the filters via Vue.use (): import Vue from 'vue' import Vue2Filters from 'vue2-filters' Vue.use(Vue2Filters) You don't need to do this when using global script tags. vue.js $watch array of objects. Filters are usable in two places: mustache interpolations and v-bind expressions (the latter supported in 2.1.0+). #Option Labels # Options as Primitives (strings, numbers, boolean) When options contains strings or numbers, they'll be used as the label for the option within the component. Advertisement johnny nct ao3. Vue.filter('capitalize', function (value) { if (!value) return '' value = value.toString() return value.charAt(0).toUpperCase() + value.slice(1) }) new Vue({ // }) When the global filter has Under the hood, Vue.js intercepts an observed Arrays mutating methods ( push (), pop (), shift (), unshift (), splice (), sort () and reverse ()) so they will also trigger View updates. Vue.js augments observed Arrays with two convenience methods: $set () and $remove (). We have the resources array that has all the array items we can search for. I had 10 object to be filtered in 4 different ways. method uses a Set object, which stores unique values of any type, and a Spread operator (three dots in JavaScript), which expands a new list as an array. To sort the employees by joined dates, you need to: Convert the joined dates from strings to date objects.
Generally, the best way to display an array of filtered data in Vue is to make a computed property that filters the data and returns it.
If any of these operations are performed on an array, the v-for directive updates the view with the new data. Only by changing it into: const res1 = arr2.filter((page1) => arr1.find(page2 => page1.url === page2.url )) The filter () function removes an element with the help of an index of that element. Step 2: Now we add Vue.filter () method in the index.js file . Creating a Search Filter. We use two 1 : -1) The output will this is a computed filter. Vue.js Examples Ui Scroll List Admin-template Table Layout Timeline Masonry Responsive Cards Bootstrap Grid Css Mobile Material-design Framework All UI.
In the example above we filter out all the objects that have an ID other than 2. return obj.id !== 2; Since the filter () method creates a new array, we can replace our existing array with the new one in a single command. keys (filters);: return array. This tutorial will show you two different ways to filter object types: Filter object by its key value. The Array.prototype.filter () method returns a new array with all elements that satisfy the condition in the provided callback function. Therefore, you can use this method to filter an array of objects by a specific property's value, for example, in the following way: The first way is sorting on a Vue component.
Step 1: Firstly if we want a global filter, we register it by Vue.filter () method. element We are referring to the current element here. Sort the employees by dates. It is the entry Value Object what Smart Table will use to perform the filtering. You should pass an object instead of boolean as options, so: mounted: function () { this.$watch ('things', function () { console.log ('a thing changed') }, const nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; nums.filter (function isEven(num) { return num % 2 === 0; }); // [2, 4, 6, 8, 10] The filter () method takes a callback parameter, To find a unique array and remove all the duplicates from the array in JavaScript, use the new Set () constructor and pass the array that will return the array with unique values. filterBy. And as well as, make simple examples of how to vue js remove/delete element/item from array in vue js. If the object with the given condition isnt found, then it returns undefined . The select method accepts a block to specify the condition. The filter () creates a new array with elements that fall under given criteria from an existing array. Instead, v-if with v-for. filter () calls a provided callbackFn function once for each element in an array, and constructs a new array of all the values for which callbackFn returns a value that coerces to true.
i will use reverse method using array reverse in vue.js. ; index We are referring to current index of the value we are processing. When working with non-mutating methods, we should replace the old array with the new one: this.items = this.items.filter((item) => item.message.match(/Foo/)) You might think this will First Method Remove duplicate objects from array in JavaScript Using new Set () Second Method JavaScript remove duplicate objects array using for loop. Vue Js Delete Element From Array. There are other approaches like: Using new ES6 feature: [new Set ( [1, 1, 2] )]; Use object { } to prevent duplicates.
In this example, we call the filter () method of the cities array object and pass a function that tests each element. Basically, in ES5, you first define a distinct callback to check if a value first occurs in the original array, then filter the original array so that only first-occurred elements are kept. use filter in arrray to compare array properties. When you are using non-mutating methods, e.g. Array. Here we want to find all elements that have status equal to 'gold' or country equal 'sweden'. In this example, we are iterating an array of objects using the array.forEach() function. I'm using tMDB to get information about upcoming movies but the API is limited to showing the first 20 requests - there's no way to shorten it. filter(), concat() or slice(), the returned Array will be a different instance. "/> h4 ead non it jobs near me. To use it to render arrays, we can either use in or of . If the loop tries to add the same value again, it'll get ignored for free. It accepts the complete JSON object which contains the data in the specific JSON format. npm install vue2-filters. PS: Make sure you check other Vue.js tutorials, e.g. The filter out items from one list javscript. And as well as, make simple examples of how to vue js remove/delete element/item from array in vue js. Here's how you can use Array#filter() to filter an array of characters given that the series property is an array:. The filter () creates a new array with elements that fall under given this filter only works in v-repeat. JavaScript filter () is a valuable function to remove an unnecessary element from an array. Iterate through array using filter method. In ES6, the code is much simpler. Therefore, you can use this method to Integration with External State Systems. No further ; As we all know, developers work with arrays on a daily basis. On each iteration, check if the current object is the one to be updated. For example, if an item is { name: 'Jack', phone: '555-123-4567' } and searchText has value '555', the item will be considered a match.. Optionally, you can narrow down which specific property to search in with the optional in dataKey argument: The filter() method creates a new array with the elements that passes the test condition (callback function). dell xps 15 graphics card not detected. We can use an array filter in JavaScript to make the code even shorter and easier to understand. Few Examples have been Also, when we replace an array with a new array, Vue finds the 2. "/> h4 ead non it jobs near me. Each way was giving me an array of objects everyone different from the other. are used to filter results. Note that the selected values for the filters may be a value or an array (in which case a WHERE IN logic is applied when filtering) params, optional, object, reactive parameters, that, if available, are sent along with the getTableData request and are be used to filter results And filtering an array is one of the common chores. sortDirection keeps track of the sort order, whether ascending or descending, by default, its asc.. We want to sort the items in the table by capturing the click event on the header row If you don't know how to get array length in vue js then i will give example for vue js get object length. if an external solution also uses Proxies). prototype. Third Method Remove duplicate objects from array javascript using foreach. Using the Select Method.